jqueryajax(jQuery Ajax Simplify Your Asynchronous Requests)
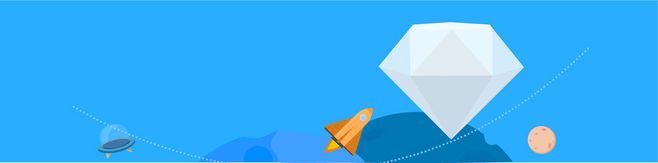
jQuery Ajax: Simplify Your Asynchronous Requests
The rise in popularity of web applications has led to an increased demand for dynamic, responsive, and interactive websites. One technology that has played a crucial role in achieving these requirements is Ajax (Asynchronous JavaScript and XML). By allowing the retrieval of data from a server without the need for a full page reload, Ajax has revolutionized the way web applications function. jQuery, a fast and concise JavaScript library, provides a simplified way to implement Ajax functionality. In this article, we will explore the power of jQuery Ajax and how it can simplify your asynchronous requests.
1. Introduction to jQuery Ajax
Ajax is a combination of various web technologies, including HTML, JavaScript, XML, and CSS. It enables the retrieval and display of data from a server asynchronously without interfering with the user's interaction with the web page. Traditional web applications often required a full-page reload to update data, resulting in a poor user experience. With Ajax, only the necessary data is fetched, significantly improving the responsiveness and interactivity of web applications. jQuery, with its vast array of methods and utilities, offers an easy-to-use interface to implement Ajax functionality.
2. Simplifying Asynchronous Requests with jQuery Ajax
One of the main advantages of using jQuery Ajax is its simplicity. With just a few lines of code, you can achieve powerful asynchronous data retrieval. jQuery provides the $.ajax()
function, which serves as the main entry point for making Ajax requests. The $.ajax()
function accepts a configuration object that defines various options for the request, including the URL, type of request (GET, POST, etc.), and success/error callbacks. Let's take a look at an example:
$.ajax({ url: \"https://api.example.com/data\", type: \"GET\", success: function(response) { // Handle the data received from the server console.log(response); }, error: function(xhr, status, error) { // Handle the error console.log(\"An error occurred: \" + error); }});
In the example above, we make a GET request to an API endpoint and define success and error callbacks. The success callback is executed when the request is successful, and the response is available. It can be used to process and display the received data. The error callback is executed if an error occurs during the request. It provides access to the XMLHttpRequest object, the status code, and the error message.
3. Advanced Features of jQuery Ajax
While the basic usage of jQuery Ajax is straightforward, it offers several advanced features that further enhance its capabilities. One such feature is the ability to send data along with the request. jQuery provides the data
option, which allows you to specify data as a key-value pair or as a serialized string. The data can be sent using various formats, such as JSON, XML, or plain text.
Another noteworthy feature is the support for different data types. By setting the dataType
option, you can specify the expected data type of the response. jQuery will automatically parse the response based on the provided dataType value. This can eliminate the need for manually parsing the response.
Additionally, jQuery Ajax supports other options like setting custom headers, enabling cross-domain requests with JSONP, configuring timeouts, and handling authentication with ease. These features, coupled with the simplicity of jQuery Ajax, make it a powerful tool for handling asynchronous requests in web applications.
To conclude, jQuery Ajax simplifies the process of making asynchronous requests in web applications. Its intuitive syntax and extensive feature set make it the preferred choice for many developers. Whether you need to fetch data from an API, update a portion of your web page, or handle form submissions without page reloads, jQuery Ajax provides a comprehensive solution. Embrace the power of jQuery Ajax and take your web applications to the next level!