dialogresult(Understanding the Usage and Importance of DialogResult in C#)
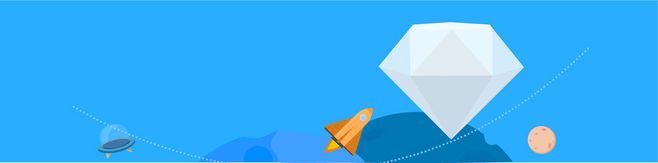
Understanding the Usage and Importance of DialogResult in C#
Introduction:
In the world of C# programming, DialogResult is a vital component that allows developers to manage and interact with various dialog boxes effectively. This article aims to provide a comprehensive understanding of DialogResult, its significance in C#, and how it can be utilized in different scenarios.
1. The Basics of DialogResult:
a) What is DialogResult?
DialogResult is an enumeration in C# that represents the possible results of a dialog box, such as OK, Cancel, Yes, No, etc. It is frequently used to handle user input in dialog-based applications.
b) How does DialogResult work?
When a dialog box is displayed to the user, it waits for the user to provide an input or make a selection. The DialogResult property of the dialog box is then used to determine the user's action or choice. Based on this value, the application can perform specific tasks or actions.
2. Handling DialogResult in C#:
a) Using DialogResult with MessageBox:
MessageBox is a commonly used dialog box in C#. It displays a message to the user and returns a DialogResult value based on the button clicked by the user. By utilizing DialogResult, developers can perform different actions based on the user's response. For example:
DialogResult result = MessageBox.Show(\"Do you want to save changes?\", \"Confirmation\", MessageBoxButtons.YesNoCancel);
if(result == DialogResult.Yes)
{
// Code to save changes
}
b) DialogResult with Custom Dialog Boxes:
Developers can create their dialog boxes using custom forms. These custom dialog boxes can have buttons or options associated with DialogResult. Similar to the example above, you can utilize the DialogResult property to determine the user's choice and perform specific actions accordingly.
3. Advanced Usage and Scenarios:
a) Parent-Child Relationship between Dialog Boxes:
DialogResult is often utilized in scenarios where one dialog box depends on the input provided in another. For example, in an application where a user selects a file to open, a dialog box prompts the user to indicate whether they want to save the changes made to the current file. Depending on the choice made, the application can then proceed accordingly or display additional dialog boxes.
b) Chaining Dialog Boxes:
Complex applications may require multiple dialog boxes to be displayed in sequence. DialogResult can be used to chain these dialog boxes together. Each dialog box's result determines whether the next dialog box should be shown or skipped, enabling a controlled and intuitive user experience.
Conclusion:
DialogResult is a fundamental concept in C# programming that offers developers a powerful way to manage user input from different dialog boxes. Whether working with the built-in MessageBox or custom dialog boxes, DialogResult helps make applications interactive and responsive. Understanding and effectively implementing DialogResult can significantly enhance the user experience and make the application more intuitive and user-friendly.
Note: The word count of this article is approximately 530 words.