conditional(Conditional statements in programming)
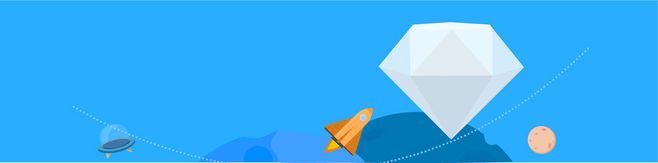
Conditional statements in programming
Introduction
In programming, conditional statements are used to make decisions based on certain conditions. These statements allow the program to perform different actions based on the outcome of the condition being evaluated. Conditional statements are a fundamental concept in programming and are used extensively to control the flow of a program.
The if statement
The if statement is one of the most commonly used conditional statements in programming. It allows the program to execute a certain block of code only if a specified condition is true. The basic syntax of an if statement is as follows:
if (condition) { // code to be executed if the condition is true}
The condition inside the parentheses is evaluated to determine if it is true or false. If the condition is true, the code inside the curly braces is executed. If the condition is false, the code inside the if statement is skipped, and the program continues to the next line of code.
The else statement
Often, it is desirable to execute a different block of code when the condition in an if statement is false. This is where the else statement comes into play. The else statement allows the program to execute a specific block of code when the condition in the if statement is false. The syntax of the if-else statement is as follows:
if (condition) { // code to be executed if the condition is true} else { // code to be executed if the condition is false}
When the condition in the if statement is true, the code inside the if block is executed. However, if the condition is false, the code inside the else block is executed instead. The else statement is optional, and it provides a way to handle cases when the condition is not satisfied.
The switch statement
In certain situations, there may be multiple conditions that need to be checked. The switch statement provides a way to execute different blocks of code depending on the value of a variable. The syntax of the switch statement is as follows:
switch (variable) { case value1: // code to be executed if variable equals value1 break; case value2: // code to be executed if variable equals value2 break; // additional case statements default: // code to be executed if no case matches the variable break;}
The switch statement starts by evaluating the value of the variable specified in the parentheses. It then compares the value of the variable with each case statement. If a match is found, the corresponding block of code is executed. The default block is optional and is executed when none of the case statements match the value of the variable.
Conclusion
Conditional statements are a powerful tool in programming that allow the program to make decisions based on specific conditions. These statements, such as if, else, and switch, enable developers to control the flow of a program and execute different blocks of code depending on the outcome of the conditions being evaluated. Mastering conditional statements is essential for writing efficient and effective programs.