eventargs(Understanding EventArgs in C# Event Handling)
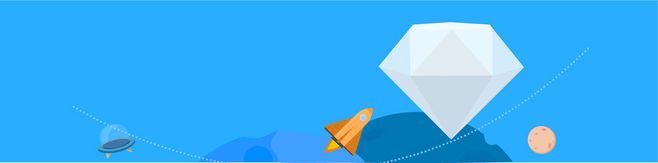
Understanding EventArgs in C# Event Handling
Introduction
In C# programming, events are an important concept that allows objects to communicate with each other. Events are usually raised by one object and handled by another object. When an event is raised, it can pass additional information to the event handler through a class called EventArgs.
What are EventArgs?
EventArgs is a base class in C# that provides a container for passing additional information about an event. It is commonly used as a parameter in event handlers to provide data associated with the event. EventArgs is a generic class that can be inherited and extended to pass custom information specific to an event.
Working with EventArgs
1. Default EventArgs
When an event does not require any additional information to be passed, the default EventArgs class can be used. This class does not have any specific properties or methods; it simply acts as a placeholder.
For example, consider a simple button click event:
```public event EventHandler ButtonClick;```The ButtonClick event does not require any additional information, so the default EventArgs class can be used:
```protected virtual void OnButtonClick(EventArgs e){ if (ButtonClick != null) ButtonClick(this, e);}```2. Custom EventArgs
Often, events need to pass some specific information to the event handlers. In such cases, a custom EventArgs class can be created by inheriting from the EventArgs base class and adding additional properties or methods.
For example, consider a custom EventArgs class for a temperature changed event:
```public class TemperatureChangedEventArgs : EventArgs{ public double NewTemperature { get; set; }}```The event handler can then be modified to use this custom EventArgs:
```public event EventHandler<TemperatureChangedEventArgs> TemperatureChanged;```The OnTemperatureChanged method can pass the new temperature as part of the custom EventArgs:
```protected virtual void OnTemperatureChanged(TemperatureChangedEventArgs e){ if (TemperatureChanged != null) TemperatureChanged(this, e);}```3. Using EventHandler delegate
The EventHandler delegate is a built-in delegate in C# that provides a generic event handler. It takes two parameters - the sender object and the EventArgs object. By using EventHandler, the event signature becomes simplified and more readable.
For example, instead of declaring the event as:
```public event EventHandler<TemperatureChangedEventArgs> TemperatureChanged;```You can simply use:
```public event EventHandler<TemperatureChangedEventArgs> TemperatureChanged;```Using EventHandler, the event handler for the TemperatureChanged event would look like this:
```void HandleTemperatureChanged(object sender, TemperatureChangedEventArgs e){ // Handle temperature change here}```Conclusion
EventArgs in C# event handling play a vital role in passing additional information from an event raiser to an event handler. Whether using the default EventArgs class or creating a custom one, EventArgs provides a flexible and extensible way to pass relevant data with events. By utilizing EventArgs effectively, C# programmers can build more robust and interactive applications.