csocket(Socket A Powerful Networking Tool in C)
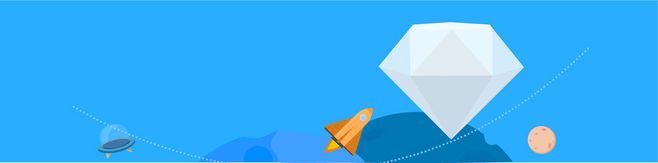
Socket: A Powerful Networking Tool in C
With the rapid development of the Internet, network programming has become an essential skill for software developers. One of the fundamental technologies underlying network programming is the socket. In this article, we will explore the concept of socket in the context of C programming language, its various functionalities, and how it can be used to build robust network applications.
Understanding Socket
A socket can be thought of as an endpoint for communication between two computers over a network. It allows two programs running on different computers to communicate by exchanging streams of data. In C, the socket is implemented as a file descriptor, which provides a convenient way to interact with the underlying network interface.
There are two types of sockets: the client socket and the server socket. The client socket initiates the connection request to the server socket, which waits for incoming connections. Once a connection is established, both sockets can send and receive data.
Socket Functionality in C
Socket programming in C involves a set of functions and data structures provided by the operating system to create, configure, and manage sockets. Let's take a closer look at some of the key functionalities offered by the socket interface in C:
Socket Creation
The first step in socket programming is to create a socket using the `socket()` system call. This function takes three arguments: the address family, the socket type, and the protocol. The address family specifies the communication domain, such as AF_INET for IPv4 or AF_INET6 for IPv6. The socket type determines the semantics of the socket, such as SOCK_STREAM for a reliable, connection-oriented socket or SOCK_DGRAM for an unreliable, connectionless socket. The protocol parameter is usually set to 0, which allows the operating system to choose the appropriate protocol for the given address family and socket type.
Binding the Socket
After creating a socket, we need to associate it with a specific address and port number using the `bind()` system call. This step is essential for server sockets to listen for incoming connections on a specific port. It also allows client sockets to specify the local address and port from which they want to establish a connection.
Listening and Accepting Connections
In order for a server socket to accept incoming connections, it needs to be put in a listening state using the `listen()` system call. The `listen()` function takes two arguments: the socket file descriptor and the maximum number of pending connections that can be queued up before the server starts rejecting new connection requests.
Once the server socket is in the listening state, it can use the `accept()` system call to accept an incoming connection request. The `accept()` function returns a new socket file descriptor representing the connection with the client. This new socket can be used to send and receive data for the specific client.
Building Network Applications with Socket
Now that we have a basic understanding of socket programming in C, let's explore how it can be used to build network applications. Here are a few examples:
Chat Application
A chat application allows multiple clients to connect to a server and exchange messages. Using socket programming, the server can listen for incoming connections and create a separate thread (or process) for each client. The clients can send messages to the server, which then distributes the messages to all connected clients.
File Transfer Application
A file transfer application enables clients to upload and download files to/from a server. With sockets, the server can listen for incoming file transfer requests and create a separate thread (or process) to handle each request. The clients can establish a connection with the server and send/receive file data over the socket.
Remote Command Execution
Socket programming also allows for remote command execution, where a client can send a command to a remote server and receive the output. The server listens for incoming command requests, executes the command, and sends the output back to the client over the socket.
Conclusion
The socket is an essential tool in network programming, allowing software developers to build powerful and scalable network applications. In this article, we discussed the concept of socket, its functionality in the C programming language, and how it can be utilized to build various network applications. By mastering socket programming in C, developers can harness the full potential of networking and create efficient and reliable software solutions.